Recently I was getting hard time to work with amazon S3 with C#. I am sharing my experience here. To start with we can create an account in http://aws.amazon.com/. It’s free to create an account here. But we have to pay for the services we want to use. There are many services available. We can choose what we need.
I had a requirement to save a file in the cloud, a json file. And periodically update the file. It’s just a storage need. So we have chosen the S3.
Now before going forward as we are supposed to do the uploading with C#, let’s first download the dot net SDK from http://aws.amazon.com/sdkfornet/ and install the SDK. This installation will give new project templates for working with cloud. After installation there will be following project templates available for development.
If we choose any of the three project types and create a project we will get a popup asking Display name, Access Key Id, Secret Access Key. To get the keys we can go to own account (click on My Account/Console Link on the top) then go to Security Credentials. If we are able to visit page we will get Access Credentials like in the image below. Here I have blacked out my credentials. We can now fill the credentials and click ok. For the name we can choose any name. VS will save this name for shortcut of the given credentials. If we visit the web config or appconfig we can see the following app keys-
If not able to visit credential page, no need to worry we will get there soon.
Now we can go to the management console. In the above image we can click AWS Management Console. We will go the console page. From the page we can choose S3. We can find it in two place- top tab and under Amazon Web Services. Both are marked in the image below. If we have created a normal account, we will not be able to do anything. We will get the message in the yellow background as in the image.
To consume this we have to signup (purchase) S3 here. As discussed earlier if we are not able to find the credentials, we will be able to find after purchase.
After we have signed up we can go to the console. And we can see two sections. Left is bucket and right is files and folders. To add any file we need to have a bucket. We can consider a bucket is nothing but a grouping of files and folders. We can group file in different folder hierarchy in the right side. To create a bucket we can chose create bucket button in the left side. We can give a name and select a region. We can create and delete a bucket from C# like this-
Create a bucket:
Delete a bucket:
I had a requirement to save a file in the cloud, a json file. And periodically update the file. It’s just a storage need. So we have chosen the S3.
Now before going forward as we are supposed to do the uploading with C#, let’s first download the dot net SDK from http://aws.amazon.com/sdkfornet/ and install the SDK. This installation will give new project templates for working with cloud. After installation there will be following project templates available for development.
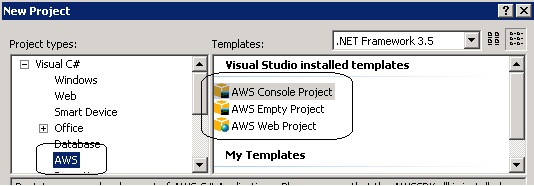
If we choose any of the three project types and create a project we will get a popup asking Display name, Access Key Id, Secret Access Key. To get the keys we can go to own account (click on My Account/Console Link on the top) then go to Security Credentials. If we are able to visit page we will get Access Credentials like in the image below. Here I have blacked out my credentials. We can now fill the credentials and click ok. For the name we can choose any name. VS will save this name for shortcut of the given credentials. If we visit the web config or appconfig we can see the following app keys-
<appSettings> <add key="AWSAccessKey" value="********************"/> <add key="AWSSecretKey" value="****************************************"/> </appSettings>Also the template will add a dll named AWSSDK.dll to the solution which contains the API library needed to communicate with S3 cloud.
If not able to visit credential page, no need to worry we will get there soon.
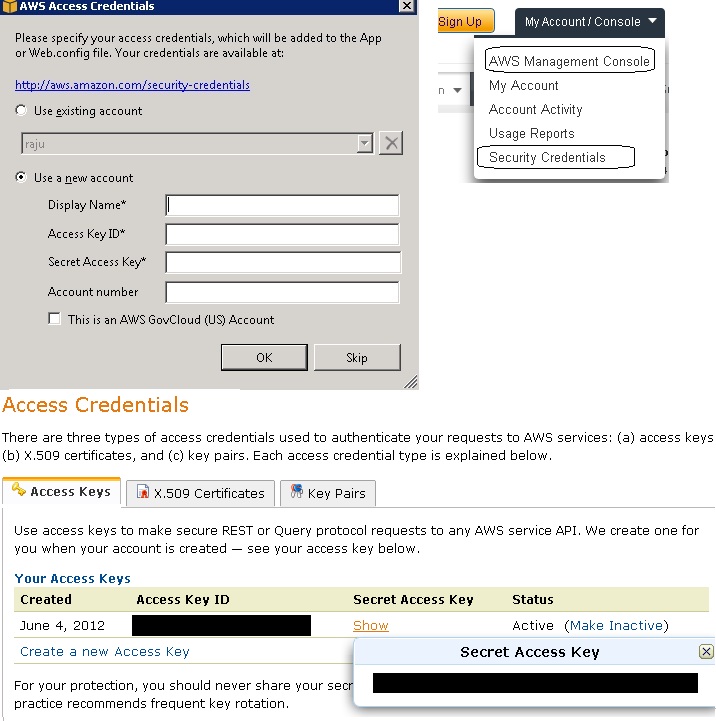%20with%20dot%20net1.jpg)
Now we can go to the management console. In the above image we can click AWS Management Console. We will go the console page. From the page we can choose S3. We can find it in two place- top tab and under Amazon Web Services. Both are marked in the image below. If we have created a normal account, we will not be able to do anything. We will get the message in the yellow background as in the image.
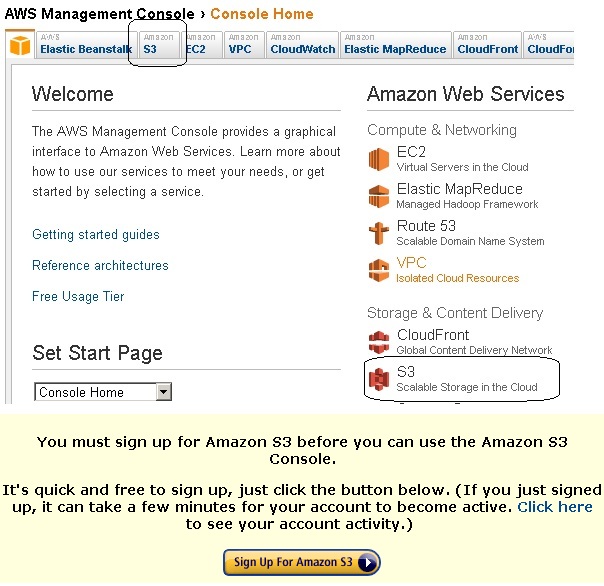%20with%20dot%20net%202.jpg)
To consume this we have to signup (purchase) S3 here. As discussed earlier if we are not able to find the credentials, we will be able to find after purchase.
After we have signed up we can go to the console. And we can see two sections. Left is bucket and right is files and folders. To add any file we need to have a bucket. We can consider a bucket is nothing but a grouping of files and folders. We can group file in different folder hierarchy in the right side. To create a bucket we can chose create bucket button in the left side. We can give a name and select a region. We can create and delete a bucket from C# like this-
Create a bucket:
Amazon.S3.AmazonS3 s3Client = Amazon.AWSClientFactory.CreateAmazonS3Client(); Amazon.S3.Model.PutBucketRequest bucket = new Amazon.S3.Model.PutBucketRequest(); bucket.BucketName = "ag_testbucket"; bucket.BucketRegion = Amazon.S3.Model.S3Region.US; s3Client.PutBucket(bucket);What we are doing here is creating an amazon S3 object. And then creating a PutBucketRequest object and using the S3 object we are creating the bucket. Similarly we can delete a bucket using DeleteBucketRequest object like below-
Delete a bucket:
Amazon.S3.AmazonS3 s3Client = Amazon.AWSClientFactory.CreateAmazonS3Client(); Amazon.S3.Model.DeleteBucketRequest bucketDelete=new Amazon.S3.Model.DeleteBucketRequest(); bucketDelete.BucketName = "ag_testbucket"; s3Client.DeleteBucket(bucketDelete);Create and update a file in cloud:
Amazon.S3.AmazonS3 s3Client = Amazon.AWSClientFactory.CreateAmazonS3Client(); try { string strBucketName="your_bucket_name"; Amazon.S3.Model.ListBucketsResponse response = s3Client.ListBuckets(); if (response.Buckets != null && response.Buckets.Where(b=>b.BucketName==strBucketName).Count()>0) { String strFileName = "test.txt"; PutObjectRequest request = new PutObjectRequest(); request.WithBucketName(strBucketName); request.WithKey(strFileName); //create data and add with the following line request.WithContentBody("sone dummy text"); PutObjectResponse objResponse = s3Client.PutObject(request); } } catch (AmazonS3Exception ex) { }To create and upload a text file using S3, we need to create an S3 client and then we have to use a bucket to upload the file. By if statement we are checking whether the bucket being used is available or not. And then we are uploading the file in the bucket.
No comments:
Post a Comment